What is method?
A Method is a group of statements that perform some task together. Method receive some information, do some process and provide a result. Method break large computing tasks into smaller ones, and enable people to build on what others have done instead of starting over form scratch. Every C# Program has at least one class with a method named Main , and all the most trivial Program can define additional functions.
Actually We are dividing our code into small and separate Modules known as Methods. This strategy allows us to think of the programming task more abstractly. Creating modules makes it easier to work with programs, allows several programmers to write or modify modules in the same programs, and enables us to create reusable programs pieces.
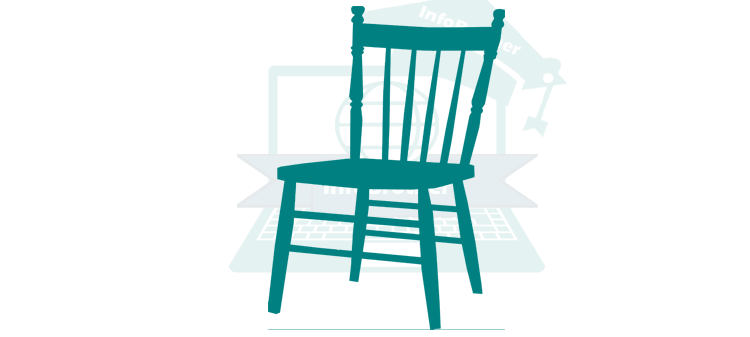
Concept:
In above chair it has a seat back and four legs. Now we need to make a seat, back and four legs out of wood. the major task is to make chair, sub tasks are, make a seat its back and then fabricate four legs. The legs should be identical. We can fashion one leg and then re-using this prototype, we have to build three more identical legs. The last task is to assemble all these to make a chair. We have a slightly difficult task and have broken down it into simpler pieces. This is the concept of functional design or top-down designing.
When we write a Method, it must start with a name, parentheses, and surrounding braces just like with main(). Methods are very important in code reusing.
There are two types of Methods:
Suppose, we have a Method that calculate the sum of two numbers such that Method will return the sum of Numbers. similarly we may have a Method which displays some information on the screen so this Method is not supposed to return any value to the calling program.
Defining Methods:
A Method Definition tells the compiler about a Method's name, return type, and parameters. The actual body of the Method can be defined separately.
In C#, every Method has a header, or Function Definition, that includes five parts:
<Access Specifier > <Return Type > <Method Name > ( Parameter List)
{
// Method Body
}
Example:
Let's have an example where we will learn how to define the method. in this example, we will define two methods in same class. the 1st method Sum will take two integer arguments and return the sum. The second method Text will be void without any arguments to display some text. both methods has Public specifier, so it can be accessed from outside the class using an instance of the class.
class Program
{
//method with parameter.
public int Sum(int x, int y)
{
int result;
result = x + y;
return result; //return x+y:
}
//method without Parameter having return type void:
public void text()
{
Console.WriteLine("Hello every one: ");
}
}
Calling Methods In C#:
We define the Method above, now its time to call or invoke the method in our Main() Program. When a Program call a method, the control is transferred to the called method. then the method will perform its task and return the control back to main method.
In order to call the method in our main program, we need to create object of containing class, then followed by .(dot) Operator we can call the method. as shown in Example:
/*Example: Methods in C#: InfoBrother:*/
using System;
namespace methods
{
class Program
{
//method with parameter.
public int Sum(int x, int y)
{
int result;
result = x + y;
return result; //return x+y:
}
//method without Parameter having return type void:
public void text()
{
Console.WriteLine("Hello every one: ");
}
//main method begin:
static void Main(string[] args)
{
int a = 20;
int b = 30;
int ret;
Program pro = new Program(); //creating object:
ret = pro.Sum(a, b); //calling 1st function:
Console.WriteLine("The sum is : " + ret);
pro.text(); //calling 2nd function:
Console.ReadKey();
}
}
}
Methods:
The sum is : 50
Hello every one:
“
After defining the method, we need to call it in our main() method to perform its operation. as we discuss already, the code define in main() method body will be execute only. the Other method could be define outside the main() method body, but we need to call it in main() Method to perform its operation:
We can also call any Public method from other classes by using the object or instance of the class. we can define the method in one class, and can call in another class using the object of that class where we define the method:
Recursive Method Call:
A method can call itself, known as Recursive Method. A Recursive usually has the two specification:
Let's have an simple example to know how this recursive method work. In this example, we will calculate factorial for a given number using a recursive method:
/*Example: Recursive Methods in C#: InfoBrother:*/
using System;
namespace methods
{
class Program
{
public static long Factorial(long Number)
{
long ReturnValue;
long NextNumber;
NextNumber = Number - 1;
if (Number == 1)
{
//This is the base case that stops the recursion.
ReturnValue = 1;
}
else
{
//This is what makes it recursion because it calls itself.
ReturnValue = Factorial(NextNumber) * Number;
}
return ReturnValue;
}
static void Main(string[] args)
{
bool ExitLoop = false;
long UsersNumber = 0;
do
{
Console.Write("Enter a number to calculate it's factorial (or 0 to exit): ");
UsersNumber = Convert.ToInt16(Console.ReadLine());
if (UsersNumber > 0)
{
if (UsersNumber > 20)
{
Console.WriteLine("Numbers entered must be between 1 and 20:");
}
else
{
//The recursion happens when the Factorial method is called.
Console.WriteLine("The Factorial of " + UsersNumber + " is "
+ Factorial(UsersNumber));
}
}
else
{
if (UsersNumber == 0) ExitLoop = true;
Console.WriteLine("The number must not be negative.");
Console.ReadKey();
}
}
while (!ExitLoop);
}
}
}
Recursive Method:
Enter a number to calculate it's factorial (or 0 to exit): 45
Numbers entered must be between 1 and 20:
Enter a number to calculate it's factorial (or 0 to exit): 14
The Factorial of 14 is 87178291200
Enter a number to calculate it's factorial (or 0 to exit): 12
The Factorial of 12 is 479001600
Enter a number to calculate it's factorial (or 0 to exit): 0
The number must not be negative.
Function Arguments:
If a function is to use arguments, it must declare variables that accept the values of the arguments. These variables are called the "formal parameters" of the function. The formal parameters behave like other local variables inside the function and are created upon entry into the function and destroyed upon exit.
While calling a function, there are three ways that arguments can be passed to function.
Call Type | Description |
---|---|
Value Parameters: | In C# all Methods arguments are passed "by value". this means that the called method is given the values of its arguments in temporary variables rather than the originals. in this case, changes made to the parameter inside the method have no effect on the argument. (read more...) |
Reference Parameters: | This method copies the reference of an argument into the formal parameter. inside the Method, the reference is used to access the actual argument used in the call. this means that changes made to the parameter affect the arguments. (read more...) |
“
Whenever we Writing a program, always keep in mind that it could be reused at some other time. also try to write in a way that it can be used to solve some other related problem. Methods are very important in case of re-usability. We can write some advance method and can use these methods in other programs. method provide us the way through which we can break our code into small pieces and make it more readable and efficient.