References:
A Criminal who uses two Names is said to have an Alias. To create a second Name for a variable in a program, we can also generate an Alias, or an alternative Name. in C#, a variable that acts as an alias for another variable is called a Reference variable, or simply a reference.
Reference is actually another Name for an already existing variable. Once a reference is initialized with a variable, either the variable Name or the reference name may be used to refer to the variable.
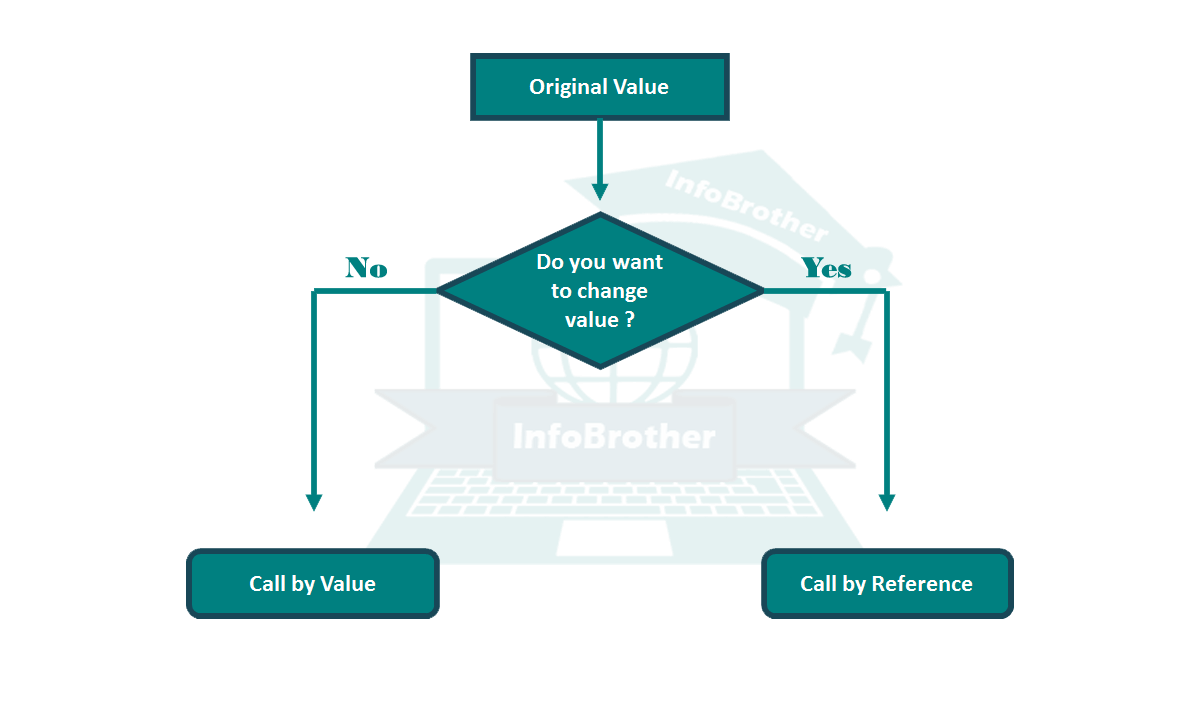
The Reference type variable holds the reference of memory address instead of Value. When we pass parameters by reference, unlike value parameters, a New storage location is not created for these Parameters. The reference parameters represent the same memory location as the Actual parameters that are supplied to the Method.
Whenever we pass a arguments by reference, The method copies the Address of an argument into the formal parameter. Inside the Method, the Address is used to access the Actual argument used in the call. This mean that changes made to the parameter affect the passed argument.
Declaring Reference Parameters:
We can Declare the Reference parameters using the ref Keyword. The following Example demonstrates this:
/*Example: Reference in C#: InfoBrother:*/
using System;
namespace reference
{
class Refer
{
//swap method:
public void swap(ref int p, ref int q)
{
int temp = p; //save the value of p;
p = q; //put q into p;
q = temp; //put temp into q;
}
//main method:
public unsafe static void Main()
{
Refer obj = new Refer();
int x = 10;
int y = 20;
Console.WriteLine("Before Swap: X:{0}, Y: {1}", x, y);
obj.swap(ref x, ref y); //method called:
Console.WriteLine("After Swap: X:{0}, Y: {1}", x, y);
Console.ReadKey();
}
}
}
Reference:
Before Swap: X:10, Y: 20
After Swap: X:20, Y: 10
Out Keyword:
The out keyword is also used to pass an argument like ref keyword, but the argument can be passed without assigning any value to it. An argument that is passed using an out keyword must be initialized in the called method before it returns back to calling method.
/*Example: Reference vs out in C#: InfoBrother:*/
using System;
namespace reference
{
class ReferVsout
{
public static void Main() //Main method
{
int val1 = 0; //must be initialized
int val2; //optional
Example1(ref val1);
Console.WriteLine(val1); // val1=1
Example2(out val2);
Console.WriteLine(val2); // val2=2
Console.ReadKey();
}
static void Example1(ref int value) //method: by Reference:
{
value = 1;
}
static void Example2(out int value) //method: by Out:
{
value = 2; //must be initialized
}
}
}
Ref Vs Out:
1
2
Ref Vs Out | |
Ref | Out |
---|---|
The parameter or argument must be initialized first before it is passed to ref. | It is not compulsory to initialize a parameter or argument before it is passed to an out. |
It is not required to assign or initialize the value of a parameter (which is passed by ref) before returning to the calling method. | A called method is required to assign or initialize a value of a parameter (which is passed to an out) before returning to the calling method. |
Passing a parameter value by Ref is useful when the called method is also needed to modify the pass parameter. | Declaring a parameter to an out method is useful when multiple values need to be returned from a function or method. |
It is not compulsory to initialize a parameter value before using it in a calling method. | A parameter value must be initialized within the calling method before its use. |
When we use REF, data can be passed bi-directionally. | When we use OUT data is passed only in a unidirectional way (from the called method to the caller method). |
Both ref and out are treated differently at run time and they are treated the same at compile time. | |
Properties are not variables, therefore it cannot be passed as an out or ref parameter. |