Interface:
Interface in C# is a Blueprint of a Class. It is like an Abstract Class Because all the methods which are declared inside the interface are abstract methods. it can't have method body and can't be instantiated.
Interfaces define Properties, Methods, and Events, which are the Members of the Interface. interface contain only the declaration of the Members and it is the responsibility of the deriving class to define the members.
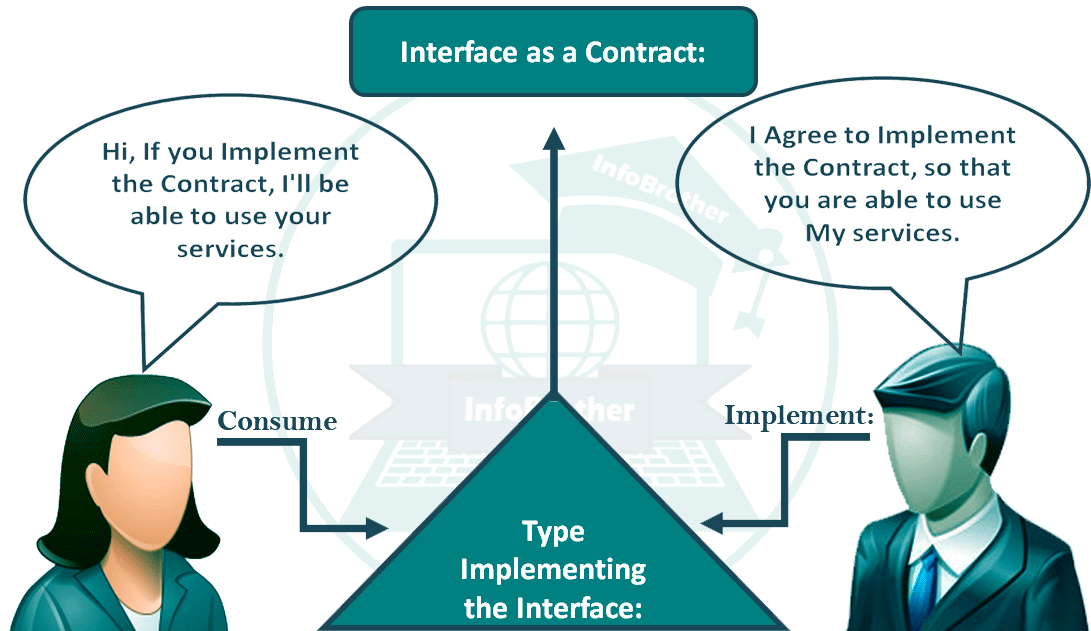
“
An interface is defined as a syntactical contract that all the classes inheriting the interface should follow. The interface defines the 'what' part of the syntactical contract and the deriving classes define the 'how' part of the syntactical contract.
Declaring Interfaces:
Interfaces are Declared using the Interface Keyword. It is Similar to Class declaration. Interface statements are Public by Default.
interface Interface_name
{
//Interface Body:
}
Let's have an simple example to know How we can declare the Interface and how to use it.
/*Example: Interface: InfoBrother*/
using System;
namespace InterFaces
{
public interface Draw //Interface Declare:
{
void drawing(); //Method Declare:
}
public class rectangle : Draw //Inherit Draw Interface:
{
public void drawing() //Method Definition:
{
Console.WriteLine("Drawing Rectangle...");
}
}
public class Circle : Draw //Inherit Draw Interface:
{
public void drawing() //Method Overridden::
{
Console.WriteLine("Drawing Circle...");
}
}
public class Square : Draw //Inherit Draw Interface:
{
public void drawing() //Method Overridden:
{
Console.WriteLine("Drawing Square...");
}
}
class Program //main class:
{
static void Main(string[] args)
{
//Object creation:
rectangle obj1 = new rectangle();
Circle obj2 = new Circle();
Square obj3 = new Square();
//calling methods:
obj1.drawing();
obj2.drawing();
obj3.drawing();
Console.ReadKey();
}
}
}
Interface:
Drawing Rectangle...
Drawing Circle...
Drawing Square...
Above, there is really simple example to understand the concept of Interfaces. in example we declare an Method Drawing() In the Interface. and define the method body in different classes using Inheritance , it was really great and simple example to know the concept of Interface.