Type Conversion:
Generally, Operators work over arguments with the same data type. However, C# has a wide variety of Data types from which we can choose the most appropriate for Particular purpose. To Perform an Operation on Variables of two different data types we need to convert both to the same data type.
As we Know C# is statically-typed at compile time, so after a variable is declared, it can't be declared again or used to store values of another type unless that type is convertible to the variable's type. For Example: There is No conversion from an Integer to any arbitrary string. therefore, after we declared and integer "x", we can't assign the string "InfoBrother" to it:
int x;
x = "InfoBrother";
// Error: "Cannot implicitly convert type 'string' to 'int' "
However, we might sometimes need to copy a value into a variable or method parameter of another type.
For Example:
We might have an integer variable that we need to pass to a method whose parameter is typed as Double. Or we might need to assign a class variable to a variable of an interface type. These kinds of operations are Called Type Conversions In C#, We can perform The following kinds of Conversion:
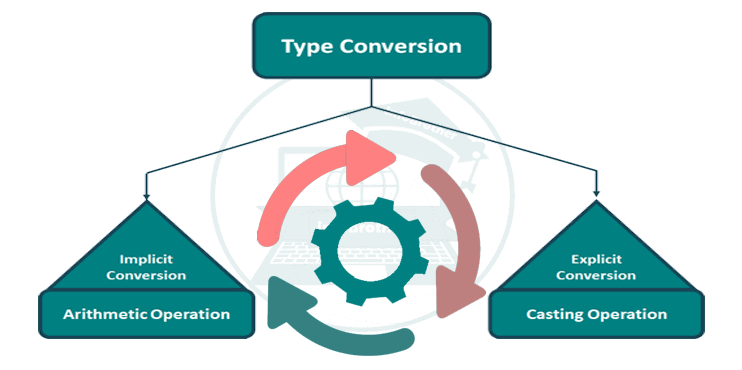
Implicit Type Conversion:
Implicit type Conversion Occurs when an Object is Assigned as the value of Variable or Method parameter. We Don't need any special Syntax for This type of conversion, because the Conversion is type safe and no data will be lost.
For Example: Int - Long , Float - double.
We can declare a variable int and assign it to a long type which is an implicit conversion as the scope of long is bigger that that of int so there is no worry of losing data.
/*Example for Implicit Conversion: InfoBrother.*/
using System;
namespace implicit_conversion
{
class Program
{
static void Main(string[] args)
{
int num1 = 40000;
int num2 = 80000;
//Now we convert int to long implicitly.
//and we don't need to tell compiler to do conversion.
//it will be done Automatically.
long total;
total = num1 + num2;
Console.WriteLine("Total is : " + total);
Console.ReadLine();
}
}
}
Implicit Conversion:
Total is : 120000
C# Supported following Implicitly type Conversions: | |
From | To |
---|---|
sbyte | short, int, long, float, double, decimal |
byte | short, ushort, int, uint, long, ulong, float, double, decimal |
short | int, long, float, double, decimal |
ushort | int, uint, long, ulong, float, double, decimal |
int | long, float, double, decimal |
uint | long, ulong, float, double, decimal |
long | float, double, decimal |
float | double |
char | ushort, int, uint, long, ulong, float, double, decimal |
Explicit Type Conversion:
In this type of conversion, we need to request explicitly to the compiler to convert one value to another by using either a Cast operator or Convert classes. A Cast is a way of explicitly informing the compiler that we intend to make the conversion and that we are aware that data loss might occur. To perform the cast, specify the type that we are casting to in parentheses in-front of the value or variable to be converted. Let's have an example to know how to perform explicit type conversion:
/*Example for Explicit Conversion: InfoBrother.*/
using System;
namespace Explicit_conversion
{
class Program
{
static void Main(string[] args)
{
double D = 32.64;
int i;
//cast double to int;
i = (int)D;
Console.WriteLine("The Value in Double is : {0}", D);
Console.WriteLine("The Value in int is : {0}", i);
Console.ReadLine();
}
}
}
Explicit Conversion:
The Value in Double is : 32.64
The Value in int is : 32
C# Provides the Following built-in type Conversion Methods:
C# Type Conversion Methods: | |
Method | Description |
---|---|
ToBoolean | Converts a type to a Boolea value, where Possible. |
ToByte | Converts a type to a byte. |
ToChar | Converts a type to a single Unicode character, where possible. |
ToDateTime | Converts a type (integer or string type) to date-time structures. |
ToDecimal | Converts a floating point or integer type to a decimal type. |
ToDouble | Converts a type to a double type |
ToInt16 | Converts a type to a 16-bit integer. |
ToInt32 | Converts a type to a 32-bit integer. |
ToInt64 | Converts a type to a 64-bit integer. |
ToSbyte | Converts a type to a signed byte type. |
ToSingle | Converts a type to a small floating point number. |
ToString | Converts a type to a string. |
ToType | Converts a type to a specified type. |
ToUInt16 | Converts a type to an unsigned int type. |
ToUInt32 | Converts a type to an unsigned long type. |
ToUInt64 | Converts a type to an unsigned big integer. |
/* Type Conversion: InfoBrother.*/
using System;
namespace Type_Conversion
{
class Program
{
static void Main(string[] args)
{
float f = 3.14f;
int i = 521457;
double d = 15002.45;
bool b = true;
//using Convert Class:
Console.WriteLine("float to double: " + Convert.ToDouble(f));
Console.WriteLine("Float to Int32: " + Convert.ToInt32(f));
Console.WriteLine("Double to Decimal: " + Convert.ToDecimal(d));
//Using Type Cast:
Console.WriteLine("Bool to String: " + b.ToString());
Console.WriteLine("Double to String: " + d.ToString());
Console.ReadLine();
}
}
}
Type Conversion:
float to double: 3.14000010490417
Float to Int32: 3
Double to Decimal: 15002.45
Bool to String: True
Double to String: 15002.45
User-Defined Conversion:
C# Enables us to declare conversion on Classes or structs so that classes or structs can be converted to and/ or from other classes or structs, or basic types. This conversion are defined like Operators and are Named for the type to which they convert. Either the type of Argument to be converted, or the type of the result of the conversion, but not both, must be the containing type.
class conversion
{
public static explicit operator Conversion(int i)
{
conversion temp = new conversion();
// code to convert from int to conversion class....
return temp;
}
}
Conversion With Classes
To Convert between Non-Compatible types, such as Integers and Datetime objects, or Hexadecimal strings and byte arrays, We can use Some conversion classes.
To convert Hexadecimal strings and byte arrays, we can use System.BitConverter class, or System.converter class.