Method Overloading:
In Our Previous Tutorial Operator Overloading we discuss about the concept of Overloading. and discuss how to overload operator. in this tutorial, we will discuss how to overload Methods and why we need to overload it.
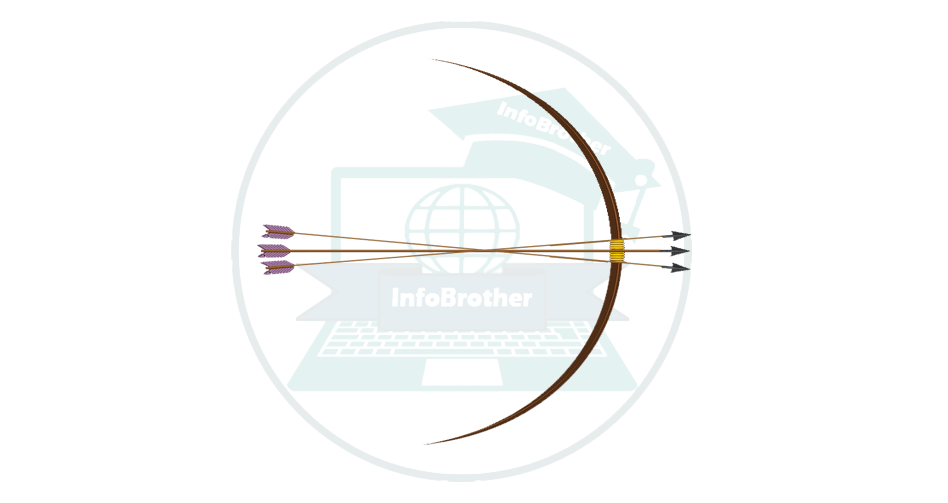
When More than one Method use the same name but with different arguments, are known as Method Overloading. it is the dominant feature of C# that allow us to use the same name for different methods to perform either same of different tasks.
Let's suppose, we need to add some numbers and we don't know exactly which numbers going to be added, it could be Int, or float, or double. So, in this case, we have 2 option. the 1st one is to write different methods with different name for each one. and then think which method has to be called, depends on the number of parameters and data type. and the other option is to create different method with same name but different parameters. and let compiler decide, which version to be called. so i guess the 2nd option is best. Let's look at the syntax that we use to overload the method.
Add(int a , int b )
{
//Method body.
}
//Overload method with different number of arguments:
Add(int a , int b , int c )
{
//Method body.
}
//Overload method with different Data type of arguments:
Add(float a , float b )
{
//Method body.
}
In above syntax, we overload same method twice with different parameters. now we will call the method and pass the parameters. the compiler will decide itself that which version to be called, depending on parameter list. let's look at simple example to know, how exactly this overloading work.
/*Example - Method Overloading - InfoBrother*/
using System;
namespace MethodOverloading
{
class Overload
{
//Method: return the sum of x and y:
public int add(int x, int y)
{
return x + y;
}
//method overload using different numbers of arguments:
public int add(int x, int y, int z)
{
return x + y + z;
}
//method overload usig different data type:
public float add(float x, float y)
{
return x + y;
}
//method overload using different data type:
public double add(double x, double y)
{
return x + y;
}
}
class Program
{
static void Main(string[] args)
{
Overload obj = new Overload();
Console.WriteLine("24 + 25 = " + obj.add(24, 25));
Console.WriteLine("24 + 25 + 16 = " + obj.add(24, 25, 16));
Console.WriteLine("2.4 + 2.5 = " + obj.add(2.4, 2.5));
Console.WriteLine("20.54 + 19.50 = " + obj.add(20.54, 19.50));
Console.ReadKey();
}
}
}
Method Overloading:
24 + 25 = 49 24 + 25 + 16 = 65 2.4 + 2.5 = 4.9 20.54 + 19.50 = 40.04
“
In case of method overloading, compiler identifies which overloaded method to execute based on number of arguments and their data types during compilation itself. Hence method overloading is an example for compile time polymorphism.