List<T> Class:
ListList<T> Class Represents a Strongly typed collection of objects which is highly optimized for providing maximum performance and can be accessed using an index. It can have duplicate elements and found in System.Collections.Generic Namespace. This class provides methods to Loop, filter, sort and Manipulate collections.
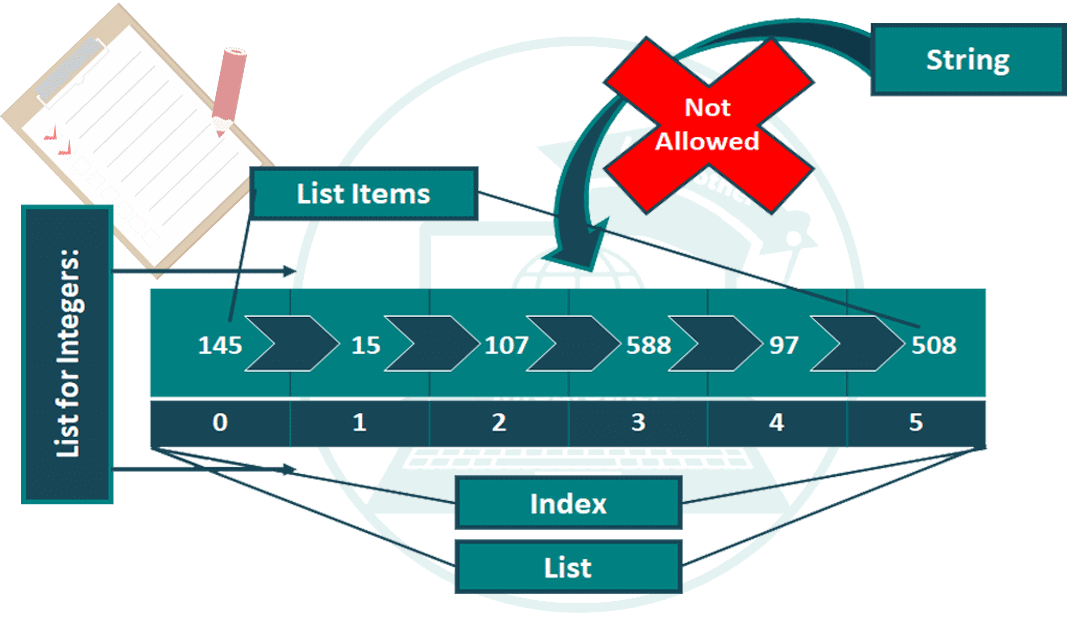
List<T> Class Provides some useful methods and properties to work with list. Following are some Commonly Used Properties of the List<T> Class.
Properties | Description |
---|---|
Capacity | Gets or sets the total number of elements the internal data structure can hold without resizing. |
Count | Gets the number of elements contained in the List<T>. |
Item[int32] | Gets or sets the element at the specified index. |
Following are some Commonly Used Methods of the List<T> Class.
Add(T) | Adds an object to the end of the List. |
---|---|
AddRange() | Adds the elements of the specified collection to the end of the List. |
BinarySearch() | Searches the entire sorted List for an element using the default comparer and returns the zero-based index of the element. |
Clear() | Removes all elements from the List. |
Contains(T) | Determines whether an element is in the List. |
ConvertAll() | Converts the elements in the current List to another type, and returns a list containing the converted elements. |
CopyTo() | Copies the entire List to a compatible one-dimensional array, starting at the beginning of the target array. |
Equals() | Determines whether the specified object is equal to the current object. |
Finalize() | Allows an object to try to free resources and perform other cleanup operations before it is reclaimed by garbage collection. |
Find() | Searches for an element that matches the conditions defined by the specified predicate, and returns the first occurrence within the entire List. |
FindAll() | Retrieves all the elements that match the conditions defined by the specified predicate. |
FindLast() | Searches for an element that matches the conditions defined by the specified predicate, and returns the last occurrence within the entire List. |
ForEach() | Performs the specified action on each element of the List. |
GetType() | Gets the Type of the current instance. |
Insert() | Inserts an element into the List at the specified index. |
Remove() | Removes the first occurrence of a specific object from the List. |
Sort() | Sorts the elements in the entire List using the default comparer. |
ToArray() | Copies the elements of the List to a new array. |
For complete list of properties and methods, please visit Microsoft's C# documentation. |
How to use List<T> Class:
» In order to use List class, first of all We need to declare the list Class Object, using this syntax:
//For Integers:
List<int> Int_List = New List<int>();
//For String:
List<String> str_List = New List<String>();
//For Student Class Objects:
List<Student> StdList = New List<Student>();
» We can Add values in List Collection using Add() Method. The following example show, how to add value in Int or string type collection.
//Add Integer value into int type List.
List<int> intList = new List<int>();
intList.Add(10);
intList.Add(20);
intList.Add(30);
intList.Add(40);
//Add String value into string type List.
List<string> strList = new List<string>();
strList.Add("one");
strList.Add("two");
strList.Add("three");
strList.Add("four");
strList.Add("four");
//Add value into the list of Object.
List<Student> studentList = new List<Student>();
studentList.Add(new Student());
studentList.Add(new Student());
studentList.Add(new Student());
» We can Retrieve the Values from List collection using foreach loop or For Loop . The following example show, how to use foreach loop to iterate a list collection.
List<int> IntLIst = new List<int>() { 10, 12, 14 };
foreach (int id in IntList)
{
Console.WriteLine(id);
}
Let's have an simple example, where we'll use different methods and properties to work with List Class. In this example, we will try to add, remove and insert values in the list.
/*Example -Collection -> List<T> Class - InfoBrother*/
using System;
using System.Collections.Generic;
public class Student : IEquatable<Student>
{
public string StudentName { get; set; } //Get and set student name:
public int StudentID { get; set; } //Get and set Student ID:
//Override Methods:
public override string ToString()
{
return "ID: " + StudentID + " Name: " + StudentName;
}
public override bool Equals(object Std)
{
if (Std == null) return false;
Student StdObj = Std as Student;
if (StdObj == null) return false;
else return Equals(StdObj);
}
public override int GetHashCode()
{
return StudentID;
}
public bool Equals(Student other)
{
if (other == null) return false;
return (this.StudentID.Equals(other.StudentID));
}
// Should also override == and != operators.
}
public class Example
{
public static void Main()
{
// Create a list of Students.
List<Student> Students = new List<Student>();
// Add Students to the list.
Students.Add(new Student() { StudentName = "Omar", StudentID = 1256 });
Students.Add(new Student() { StudentName = "usman", StudentID = 1255 });
Students.Add(new Student() { StudentName = "Khizar", StudentID = 1254 });
Students.Add(new Student() { StudentName = "Taimur", StudentID = 1252 });
Students.Add(new Student() { StudentName = "Sana javed", StudentID = 1253 });
Students.Add(new Student() { StudentName = "Noor", StudentID = 1251 });
/* Write out the Students in the list. This will call the overridden
* ToString method in the Student class. */
//Retrieve Items from the list:
Console.WriteLine("Student Record:");
foreach (Student aPart in Students)
{
Console.WriteLine(aPart);
}
/*Check the list for Student ID #1255. This calls the IEquitable.Equals method
of the Student class, which checks the Student ID for equality. */
Console.WriteLine("\nDo We Have Student ID # 1255 ?: {0}",
Students.Contains(new Student { StudentID = 1255, StudentName = "" }));
Console.WriteLine("Do We Have Student ID # 1235 ?: {0}",
Students.Contains(new Student { StudentID = 1235, StudentName = "" }));
// Insert a new item at position 2.
Console.WriteLine("\nInsert new Student ID # 1255 At location # 3");
Students.Insert(3, new Student() { StudentName = "Kinat", StudentID = 1225 });
//Retrieve Items from the list:
Console.WriteLine("Student Record:");
foreach (Student aPart in Students)
{
Console.WriteLine(aPart);
}
Console.WriteLine("\nAt Index[3] We have: {0}", Students[3]);
//Remove Student from specific position of list:
Console.WriteLine("\nRemove student ID # 1253 from list:");
/* This will remove Student ID 1253 even though the Student Name is different,
because the Equals method only checks Student ID for equality. */
Students.Remove(new Student() { StudentID = 1253, StudentName = "Farhan" });
//Retrieve Items from the list:
Console.WriteLine("Student Record:");
foreach (Student aPart in Students)
{
Console.WriteLine(aPart);
}
Console.WriteLine("\nRemove Student from Index[3]:");
// This will remove the Student at index 3.
Students.RemoveAt(3);
//Retrieve Items from the list:
Console.WriteLine("Student Record:");
foreach (Student aPart in Students)
{
Console.WriteLine(aPart);
}
Console.ReadKey();
}
}
List<T> Class:
Student Record: ID: 1256 Name: Omar ID: 1255 Name: usman ID: 1254 Name: Khizar ID: 1252 Name: Taimur ID: 1253 Name: Sana javed ID: 1251 Name: Noor Do We Have Student ID # 1255 ?: True Do We Have Student ID # 1235 ?: False Insert new Student ID # 1255 At location # 3 Student Record: ID: 1256 Name: Omar ID: 1255 Name: usman ID: 1254 Name: Khizar ID: 1225 Name: Kinat ID: 1252 Name: Taimur ID: 1253 Name: Sana javed ID: 1251 Name: Noor At Index[3] We have: ID: 1225 Name: Kinat Remove student ID # 1253 from list: Student Record: ID: 1256 Name: Omar ID: 1255 Name: usman ID: 1254 Name: Khizar ID: 1225 Name: Kinat ID: 1252 Name: Taimur ID: 1251 Name: Noor Remove Student from Index[3]: Student Record: ID: 1256 Name: Omar ID: 1255 Name: usman ID: 1254 Name: Khizar ID: 1252 Name: Taimur ID: 1251 Name: Noor