References:
A Criminal who uses two Names is said to have an Alias. To create a second Name for a variable in a program, we can also generate an Alias or an alternative Name. in C++, a variable that acts as an alias for another variable is called a Reference variable, or simply a reference.
Reference is actually another Name for an already existing variable. Once a reference is initialized with a variable, either the variable Name or the reference name may be used to refer to the variable.
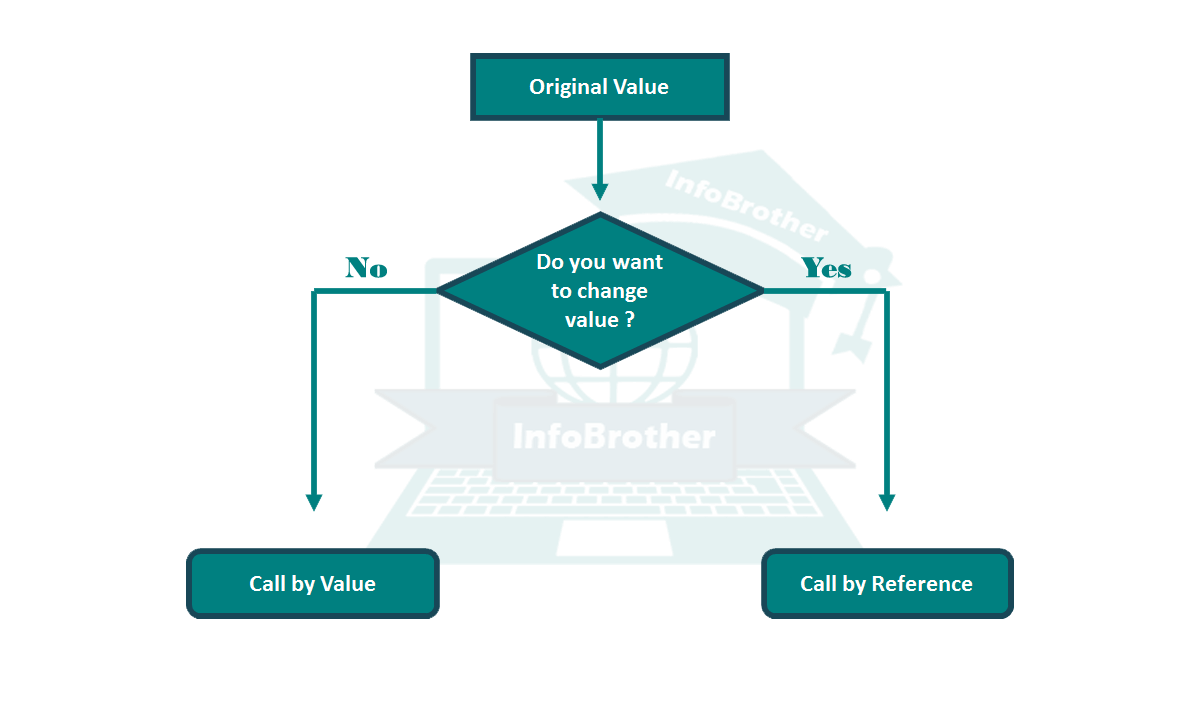
Pointer Vs Reference:
In C++ references provide many of the same capabilities as pointer, Although most C++ programmers seem to develop some intuition about when to use references instead of pointers and vice versa, they still encounter situations where the choice isn't so clear. References are often confused with pointers but three major differences between references and pointers are:
Declaration of References:
A reference declaration has essentially the same syntactic structure as a pointer declaration. The difference is that while a pointer declaration uses the * operator, a Reference declaration uses the & operator. For Example:
int x = 10;
int *ptr = & x;
First we declare an variable "x" and store some value in it. and in second step we declare an pointer "ptr" and initialize it with the address of variable "x". on the other hand:
int x = 10;
int &ref = x;
We Declare "ref" as a variable of type Reference to int referring to x. the difference between the pointer and reference declaration is Noteworthy, but it's not the basis for deciding to use one over the other. the real basis for the choice is the difference in appearance between references and pointers when we use them in expressions.
Reference Parameters
while it is possible to achieve a call-by-reference manually by using the pointer operators, this approach is rather clumsy. First, it compels you to perform all Operations through pointers. second, it requires that you remember to pass the addresses (rather than the values) of the arguments when calling the function.
Fortunately, in C++, it is possible to tell the compiler to automatically use call-by-reference rather than call-by-value for one or more parameters of a particular function. We can accomplish this with a reference parameter. when we use a reference parameter, the address (not the value) of an argument is automatically passed to the function. within the function, operations on the reference parameter are automatically dereferenced, so there is no need to use the pointer operators.
Let's have a simple example to understand, how we can pass arguments by reference to function.
/*call by Reference: InfoBrother*/
#include <iostream>
using namespace std;
//function, used to change the value of actual variable:
void increment(int &x)
{
++x; //increment the value.
}
main()
{
int x=10; //actual variable.
int &ptr = x; //create an reference variable.
cout<<" Before increment: "<<x<<endl;
increment(x); //function calling. call by reference:
cout<<" After increment: "<<x<<endl;
return 0;
}
Argument passing by Reference:
Before increment: 10
After increment: 11
As apparent from the Name By Reference we are not passing the value itself but some form of reference or address. To understand this, we can think in terms of variables which are names of Memory locations. We always access a variable by its Name (which in fact is accessing a Memory location), a variable name acts as an address of the Memory location of the variable.
If we want the called function to change the value of a variable of the calling function, we must pass the address of that variable to the called function. Thus, by passing the address of the variable to the called function, we convey to the function that the number you should change is lying inside this passed memory location, square it and put the result again inside that memory location. When the calling function gets the control back after calling the called function, it gets the changed value back in the same memory location. In summary, while using the call by the reference method, we can’t pass the value. We have to pass the memory address of the value.
Advantages & Disadvantages of Reference:
By using References, we are Actually passing the address of variable to Function. Passing an Address of a variable to a function has a Number of Advantages and Disadvantages:
“
In C++, we can pass Arguments to function by three different ways, The first and Default way is "Pass by value" and second is "pass by pointer" and the third is "pass by reference". we already discussed "call by value and call by pointer" in previous tutorial (C++ Pointers). in Next tutorial, we will discuss more "Passing Arguments".