What is Multi-threading?
Multi-threading or Multitasking is an art of handling different (More than one) task simultaneously at a given point of time. also called as Multi-processing:
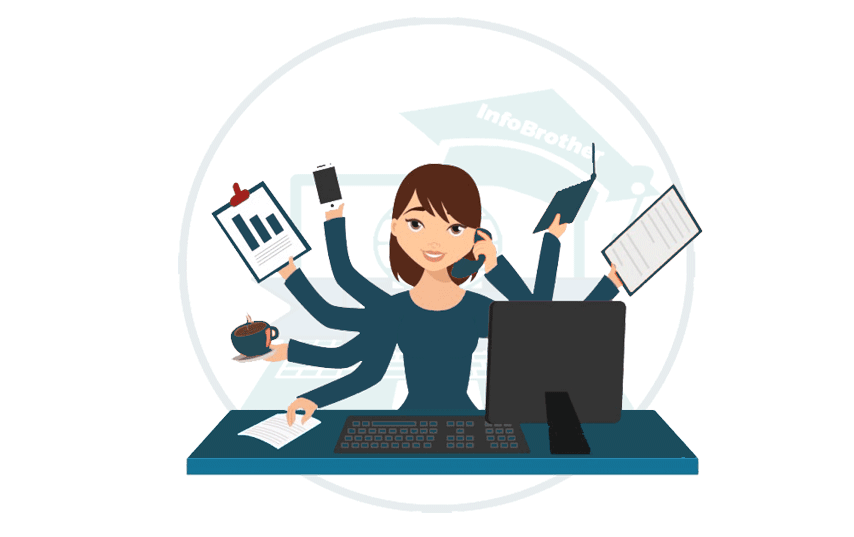
It’s no secret that for many jobs multitasking is an essential requirement. A common example of multitasking at work is a representative juggling numerous tasks at once like talking on the telephone, taking notes and checking emails at the same time. we all have multitasking for each minute like we talk on the phone while driving, text while keeping conversation with friends, and constantly check emails while watching favourite shows etc.
Multi-threading in C++:
“
A programmable approach to achieve multi-tasking in known as multi-threading. A multi-threaded program contains two or more parts that can run concurrently. Each part of such a program is called a thread, and each thread defines a separate path of execution.
The concept of the Multi-threading comes from the multitasking. And, what is multitasking? As we discuss, it allows our system to execute many programs concurrently. If we see the details of the multitasking, we will find that there are two kinds of multitasking known as thread-based and process-based process-based. Process-based multitasking refers to running many programs concurrently. Thread-based multitasking, however, refers to running the fragments of the same program.
. A multi-threaded program, in fact, has many parts that can be executed concurrently. So, a part of the program is known as the thread. Each thread offers the path of the execution. Well, multi-threaded application is not supported in the C++. Instead, it is dependent on the OS we are using. If we have created the virtual machines, then it can also rely on those.
The reason behind the use of the multi-threading is that it increases the flexibility and the efficiency of our programs.
Why we need Multi-threading in C++?
Threads are the popular way to improve application through parallelism. For example, in a browser, multiple tabs can be different threads. MS word uses multiple threads, one thread to format the text, other thread to process inputs, etc. Threads operate faster than processes due to following reasons:
Creating Threads:
Before getting start to creating threads, we need to include a Library: <thread>
Let's have an example here to know how this Multi-threading work in C++:
/*Multi-threading Example: Infobrother: */
#include <iostream>
#include <thread>
using namespace std;
void function()
{
cout<<"Hello world: "<<endl;
}
main()
{
//call function using thread Object:
thread call_function(funtion);
return 0;
}
In above example, we create an object of thread Call_function having parameter function.
When we try to compile this program, an Runtime Error will generate. because the main thread does not wait for Call_function thread termination. It continues its work. The main thread finishes execution, but Call_function is still running. So an error occur. All the threads must be terminated before main thread is terminated.
How to solve it?
To Avoid this Runtime error in our program, we need to join the threads. when we join the thread, the main thread will wait until child thread does not finish its execution. Before joining, The main thread finish Its execution and does not wait for the child thread, while In case of joining threads, the main thread has to wait for child thread.
How to join thread?
We can join thread using join() member function of a thread class. so after joining our program will look like this:
/*Multi-threading Example: Infobrother: */
#include <iostream>
#include <thread>
using namespace std;
void function()
{
cout<<"Hello world: "<<endl;
}
main()
{
//call function using thread Object:
thread call_function(function);
//main thread block, until child thread execution finish:
call_function.join();
return 0;
}
Multi-threading
Hello world:
Now our Program executed successfully. but after join() returns, the Threads will become not join-able.
So its always better to check if the thread is join-able or not. We can check if a thread is join-able or not by using, joinable() member function of class thread.
/*Multi-threading Example: Infobrother: */
#include <iostream>
#include <thread>
using namespace std;
void function()
{
cout<<"Hello world: "<<endl;
}
main()
{
//call function using thread Object:
thread call_function(function);
if(call_function.joinable())
{
//main thread block, until child thread execution finish:
call_function.join();
}
else
cout<<" Call_function is not join-able at the moment: "<<endl;
return 0;
}
Passing Arguments to Thread:
In Our Previous examples: we just used functions and objects without passing any arguments to these function and objects. but we can use a function with parameters to thread initialization. Let's check an example to know how to do that.
/*Example: Passing arguments to thread: Infobrother:*/
#include <iostream>
#include <thread>//required for threads operation:
#include <chrono>//required for time operation (sleep);
using namespace std;
void functionA(int thread_number, int iterations, long delay)
{
int loop = 0;
// Loop some 'iterations' number of times
while(loop < iterations)
{
// Sleep for some time
this_thread::sleep_for(std::chrono::milliseconds(delay));
cout << "Thread " << thread_number << " Reporting: " << loop
<< " with delay " << delay <<endl;
loop++;
}
}
int main()
{
char result;
// Launch two new threads. They will start executing immediately
// functionA is a generic worker that will take in two parameters:
// Number of iterations, and Sleep time
thread thread1(functionA, 1, 10, 1555);
thread thread2(functionA, 2, 10, 2222);
// Pause the main thread
cout << "Press a key to finish" <<endl;
cin >> result;
// Join up the two worker threads to the main thread
thread1.join();
thread2.join();
// Return success
return 0;
}
Passing Arguments to Thread:
Press a key to finish
Thread 1 Reporting: 0 with delay 1555
Thread 2 Reporting: 0 with delay 2222
Thread 1 Reporting: 1 with delay 1555
Thread 2 Reporting: 1 with delay 2222
Thread 1 Reporting: 2 with delay 1555
Thread 1 Reporting: 3 with delay 1555
Thread 2 Reporting: 2 with delay 2222
Thread 1 Reporting: 4 with delay 1555
Thread 2 Reporting: 3 with delay 2222
Thread 1 Reporting: 5 with delay 1555
Thread 1 Reporting: 6 with delay 1555
Thread 2 Reporting: 4 with delay 2222
Thread 1 Reporting: 7 with delay 1555
Thread 2 Reporting: 5 with delay 2222
Thread 1 Reporting: 8 with delay 1555
Thread 2 Reporting: 6 with delay 2222
Thread 1 Reporting: 9 with delay 1555
Thread 2 Reporting: 7 with delay 2222
Thread 2 Reporting: 8 with delay 2222
Thread 2 Reporting: 9 with delay 2222
Advantage of Multithreading:
If our computer's hardware offers two processor, then two threads can run simultaneously. and even on a uni-processor, multi-threading can offer an advantage. Most Programs can't perform very many statements before they need to access the hard disk. this is a very slow operation. and hence the Operating system puts the program to sleep during the wait. In fact, the Operating system assigns the computers hardware resources to somebody else's program during the wait. But, if we have written a Multi-threaded program, then when one of our thread stalls, our other threads can continue.