Encapsulation:
Encapsulation is a Programming Mechanism that binds together code and the data it manipulates, and that keeps both safe from outside interference and misuse. In an object-oriented language, code and data can be bound together in such a way that a self-contained black box is created. Within the box are all Necessary data and code. When code and data are linked together in this fashion, an object is created. In others words, an object is a device that supports encapsulation.
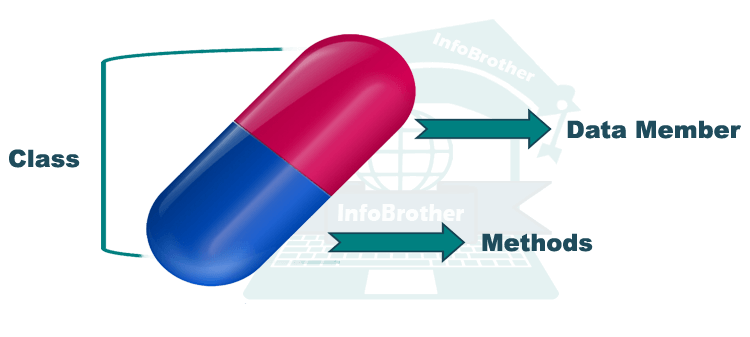
All C++ Programs are composed of the following two fundamental elements: Program Methods or Function and Program Data.
Encapsulation is an Object Oriented Programming concept that binds together the data and functions that manipulate the data, and that keeps both safe from outside interference and misuse.
Data Encapsulate in C++:
If Someone hands us a Class, we don't need to know how it actually works to use it, all we need to know about its public methods/data Its Interface . This is often compared to Operating a Car: When we drive, we don't care how the steering wheel makes the wheels turn, we just care that the interface the car Presents (The Steering Wheel ) allow us to accomplish our goal. Interfaces abstract away the details of how all the Operations are actually performed, allowing the Programmer to focus on how objects will use each other's interfaces, how they interact.
This is why C++ Makes our specify Public and Private Access Specifiers: by Default, it assumes that the things we define in a Class are internal details which someone using our code should not have to worry about. The Practice of hiding away these details from client code is called " Data Hiding " , or Making our class a "Black Box".
C++ Supports the Properties of Encapsulation and data hiding through the creation of user-define types, called Classes. We already know that a class can contain Private, Protected, and Public Members. By Default, all items defined in a class are private. For Example:
class addition //class begin
{
private: //Private Members: (data Member)
int UpperLimit;
int sum;
int Number;
public: //Public Members: (Method)
addition()
{
//body
}
void showResult()
{
//body
}
};
The Variable UpperLimit, sum, and Number are Private. This means that they can be accessed only by other Members of the addition class, and not by any other part of our Program. This is one way encapsulation is achieved.
To Make Parts of a Class Public ( i.e. Accessible to other parts of our program), We must declared them after Public Keyword. All Variables or functions defined after the public specifier are accessible by all other functions in our program. In the above example "Data Abstraction" abstract the data from outside the class, while "Data Encapsulation" Bundling the data and function.
/* Data Encapsulation: this Program can return you sum of all numbers till
UpperLimit. e.g. Upperlimit is 5, then it will return 5+4+3+2+1+0=15 */
#include<iostream>
using namespace std;
class addition
{
private:
int UpperLimit; //to get Upper Limit from User.
int sum; //will use to store the Sum of Numbers.
int Number; //all Numbers less than upperlimits.
public:
addition()
{
UpperLimit=0;
sum=0;
Number=0;
}
void showResult()
{
cout<<" Enter the Upper Limit to get sum: ";
cin>>UpperLimit;
/*Loop will be continue until reach at UpperLimit Number.*/
while(Number<UpperLimit) //Number is less than UpperLimit.
{
Number=Number+1; //Increment number one by one.
sum=sum+Number; /*All numbers will added to sum and will
store in sum variable.*/
//Example. sum=0+1=1; sum=1+1=2 and so on.
}
//when the condition has gone false, mean Number will reach upperlimit.
cout<<"The sum of first "<<UpperLimit
<<" Number are: "<<sum;
}
};
main()
{
addition obj;
obj.showResult();
return 0;
}}
Data Encapsulation
Enter the Upper Limit to get sum: 25
The sum of first 25 Number are: 325
“
One Way to Think about What happens in an Object-Oriented-Program is that we define what objects exist and what each one knows, and then the objects send messages to each other (by Calling each other's methods) to Exchange information and tell each other what to do.