Exception Handling:
Sometimes, function not working properly even mine. Most beginning Programmers assume that their programs will work as Expected.
On the other side, Experienced programmers know that things go unexpected. if we issue a command to read a file from a disk, the file might not exist. or if we want to write to a disk, the disk might be full or un-formatted. if the programs asks for user input, users might enter invalid data.
Such errors that occur during the Execution of Object Oriented Programming are called Exceptions. and the techniques to manage such errors comprise the group of method known as Exception Handling.
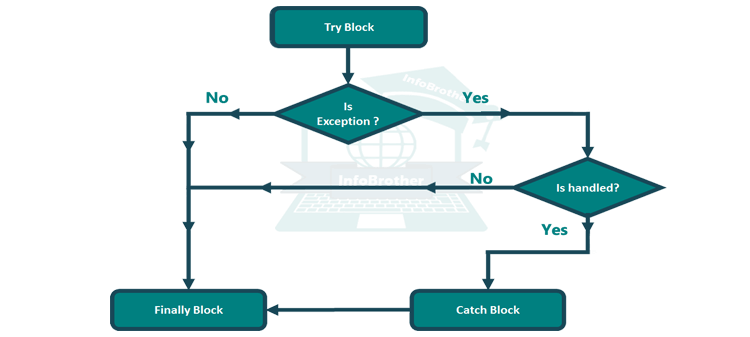
C++ Exception handling is built upon three keywords: try, Catch and throw. The General idea is that code which has the Potential to go wrong in some way is wrapped in a try block, using the throw keyword when it encounters an exception, and then one of the catch blocks which follows the inline block can handle the exception in some way. A good example to demonstrate the use of this might be a simple application which divides two numbers, like the following.
#include<iostream>
using namespace std;
int number(int a, int b)
{
return a/b;
}
main()
{
int x, y;
cout<<"Enter two numbers: "<<endl;
cin>>x>>y;
cout<<number(x,y);
return 0;
}
The above would perfectly fine, but what about if the user entered "b" as "0", since dividing by 0 is "IMPOSSIBLE" (I think so...) This answer is that it would probably give some kind of Runtime error or exception. in a "real world" application, the user probably wouldn't know what this meant and it'd be awfully confusing for everybody involved in the process of trying to help fix the problem. A good way to deal with this might be to use try, Catch and throw.
Try:
A try block identifies a block of code for which particular exceptions will be activated. it is followed by one or more catch blocks. The try block must contain the portion of our program that we want to monitor for errors. This section can be as short as a few statements within one function, or as all-encompassing as a try block that encloses the main() function code. (which would, in effect, cause the entire program to be monitored).
throw:
A program throws an exception when a problem shows up. This is done using a throw keyword. The general form of the throw statement is: throw exception; throw generates the exception specified by exception. if this exception is to be caught, then throw must be executed either from within a try block itself, or from any function called from within the try block, whether directly or indirectly.
catch:
A Program catches an exception with an exception handler at the place in a program where we want to handle the problem. The catch keyword indicates the catching of an exception. there can be more than one catch statement associated with a try block. The type of the exception determines which catch statement is used. that is, if the data type specified by a catch statement matches that of the exception, then that catch statement is executed (and all other are bypassed)
“
if an exception is thrown for which there is no applicable catch statement, an abnormal program termination will occur. this is, our program will stop abruptly in an uncontrolled manner. thus, we will want to catch all exception that will be thrown.
The Syntax is:
try
{
//try block:
throw exception;
}
catch(type exception)
{
//code to be executed in case of exception.
}
The code within the try block is executed normally. In case that an execution takes place, this code must use the throw keyword and a parameter to throw an exception. the type of the parameter details the exception and can be of any valid type. if an exception has taken place, that is to say, if it has executed a throw instruction within the try block, the catch block is executed receiving as parameter the exception passed by throw . let's have an example here: the following simple program will show you the concept of try, catch and throw block. the output of program explains flow of execution of try/catch blocks:
/*Exception Handling: InfoBrother:*/
#include<iostream>
using namespace std;
main()
{
/*Enter the value of x: if x>0, program execute normally:
if x<0: the program will catch the error and display the error:*/
int x;
cout<<" Enter the value of x: ";
cin>>x;
cout<<" Before try: "<<endl;
try
{
cout<<" Inside try: "<<endl;
if(x < 0) //check the code here:
{
throw x; //if x<0, throw x:
//after throw statement: nothing will execute in try block ever:
cout<< "After throw: "<<endl; //will not execute ever:
}
}
catch (int x) //if try throw any exception, catch it:
{
//if the program will catch exception: then the code inside this block will execute:
cout<<" Exception Caught: "<<endl;
//handle the exception here,
}
cout<<" After catch: "<<endl;
return 0;
}
Exception Handling
Enter the value of x: 5
Before try:
Inside try:
After catch:
Enter the value of x: -1
Before try:
Inside try:
Exception Caught:
After catch:
Enter the value of x: 0
Before try:
Inside try:
After catch:
C++ Standard Exceptions:
C++ provides a list of standard exception defined in <exception> which we can use in our programs. Figure below shows the relationship of these classes to the exception class. Two of the first generation descendants of exception are logic_error and runtime_error, each of these has several descendants of its own.
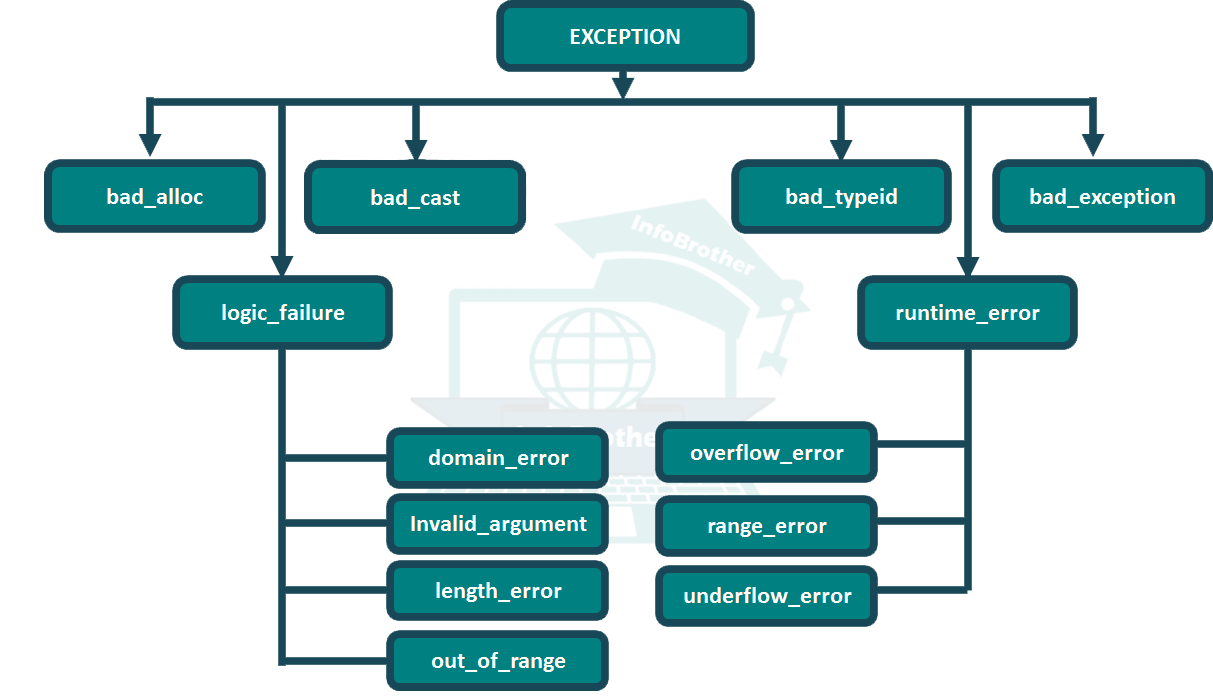
“
To use these functions in our program, we need to include <exception> header file at the top of our program:
And here is the brief explanation of all these Exception:
Dynamic_cast: Its an operator, which can be used to convert safely from a pointer, or reference to a base type to a pointer or reference to a derived type.
typeid Operator: typeid is an operator which return the actual type of the object referred to by a pointer or a reference.
C++ Standard Exceptions: | |
Exception | Description |
---|---|
bad_alloc | bad_alloc is the type of the object thrown as exception by the allocation function to report failure to allocate storage. This can be thrown by New Operator while allocating dynamic memory. |
bad_cast | An exception of this type is thrown when a Dynamic_cast to a reference type fails the run-time check ( because the types are not related by inheritance) |
bad_typeid | An exception of this type is thrown when a typeid typeid Operator operator is applied to a dereferenced null pointer value of a polymorphic type. |
bad_exception | bad_exception is the type of exception thrown by the C++ runtime to handle unexpected exceptions in a C++ program. |
logic_error | Defines a type of object to be thrown as exception. it reports error that are a consequence of faulty logic within the program such as violating logical preconditions or class invariant and may be preventable. |
domain_error | Defines a type of object to be thrown as exception. it may be used by the implementation to report domain errors, that is , situations where the inputs are outside of the domain or which an operation is defined. |
invalid_argument | Defines a type of object to be thrown as exception. it reports errors that arise because an argument value has not been accepted |
length_error | Defines a type to be thrown as exception. it reports errors that are consequence of attempt to exceed implementation defined length limits for some object. this function is thrown when a too big string is created. |
out_of_range | Defines a type of object to be thrown as exception. it reports errors that are consequence of attempt to access elements out of defined range. this can be thrown by the 'at' method. (For example: std::vector and std::bitset). |
runtime_error | Defines a type of object to be thrown as exception. it reports errors that are due to events beyond the scope of the program and can not be easily predicted. |
overflow_error | Defines a type of object to be thrown as exception. it can be used to report arithmetic overflow errors. (that is: situations where a result of a computation is too large for the destination type) |
range_error | Defines a type of object to be thrown as exception. it can be used to report range errors ( that is: situation where a result of a computation cannot be represented by the destination type). |
underflow_error | Defines a type of object to be thrown as exception. it may be used to report arithmetic underflow errors, (that is: situation where the result of a computation is a subnormal floating-point value) |
Define New Exceptions:
We can define our own exceptions by inheriting and overriding exception class functionality. Following is the example, which shows how we can use exception class to implement our own exception in standard way:
/*Define New Exception: InfoBother:*/
#include <iostream>
#include <exception>
using namespace std;
struct MyException : public exception
{
const char * what () const throw ()
{
return "C++ Exception";
}
};
main()
{
try
{
throw MyException();
}
catch(MyException& e)
{
cout << "MyException caught" <<endl;
cout << e.what() <<endl;
}
catch(exception& e)
{
//Other errors
}
return 0;
}
Define New Exception
MyException caught
C++ Exception
Here, what() is a public method provided by exception class and it has been overridden by all the child exception classes. This returns the cause of an exception.