Namespace:
Let's suppose, we are two Friends with the same Name, "Omar", in the same class. Now Whenever Our mates need to differentiate us, they would have to use some additional Information ( Like: our father Name, or Usually the "Nick" name etc. ).
Same things could be happen with our C++ Programs. Like: we write a function sort() in the class. and also we include a Library Having the same function sort() with different implementation. Now the compiler or linker will produce an error, because it does not have enough information to know that which version of sort() function are refereeing to within our code.
So, to overcome this difficulty, C++ allow us to declare our own namespace using Namespace Keyword.
A Namespace is a declarative region that provides a scope to the identifiers ( the name of types, functions, variables, etc) inside it. Namespace are used to organize code into logical groups and to prevent name collisions that occur especially when we include multiple Libraries in our program. All identifiers at namespace scope are visible to one another without Qualification. and identifiers outside of the namespace can access the members by using the fully Qualified name for each identifier.
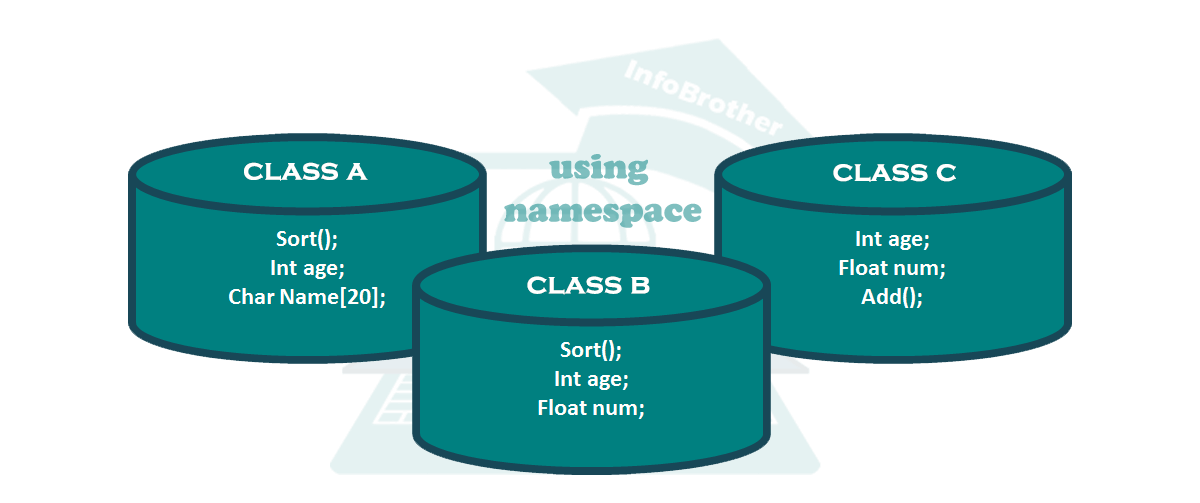
Defining Namespace:
Namespace definition begin with the Keyword "namespace" same like defining a class.
namespace identifier
{
//namespace body
}
namespace add
{
int a, b;
}
In Above Example, a and b are normal variables integrated within the add namespace.
Nested: We can define Namespace within another namespace, known as nested namespace.
Alias: Creating second name for a variable known as alias or alternative name, In C++, a variable that acts as an alias for another variable is called a "reference variable", or simply a "reference".
Using Namespace:
There are three Ways to use a Namespace in Program:
» Using Scope Resolution Operator
» Using Directive
» Using Declaration
Using Scope Resolution Operator:
Elements belonging to a namespace can be referenced directly by name within the namespace. if we need to reference an element from outside of the namespace, we must supply the namespace. To do so, we can use scope resolution Operator ( :: ) before the element name.
For Example:
code::x = 15; //outside of stuff:
/* Namespace using Scope Resolution:*/
#include<string>
#include<iostream>
using namespace std;
namespace MySpace // namespace std
{
std::string mess = "Within namespace MySpace";
int count = 0; // Definition: MySpace::count
double f( double); // Prototype: MySpace::f()
}
namespace YourSpace //another namespace.
{
std::string mess = "Within namespace YourSpace";
void f( ) // Definition of YourSpace::f()
{
mess += '!';
}
}
namespace MySpace // Back in MySpace.
{
int g(void); // Prototype of MySpace::g()
double f( double y) // Definition of MySpace::f()
{
return y / 10.0;
}
}
int MySpace::g( ) // Separate definition of MySpace::g()
{
return ++count;
}
main()
{
cout << "Testing namespaces!\n\n"
<< MySpace::mess <<endl;
MySpace::g();
cout << "\nReturn value g(): " << MySpace::g()
<< "\nReturn value f(): " << MySpace::f(1.2)
<< "\n---------------------" <<endl;
YourSpace::f();
cout << YourSpace::mess <<endl;
return 0;
}
Namespace Using Scope Resolution Operator:
Testing namespaces!
Within namespace MySpace
Return value g(): 2
Return value f(): 0.12
---------------------
Within namespace YourSpace!
Using Directive:
Using keyword allows us to import an entire Namespace into our program with a global scope. It ca be used to import a namespace into another namespace or any program.
Example:
using namespace code;
This statement allows us to reference the identifiers in the code namespace directly, if code contains an additional namespace and a using directive, this namespace is also imported.
/*Namespace using Directive*/
#include <iostream>// Namespace std
void message() // Global function ::message()
{
std::cout << "Within function ::message()\n";
}
namespace A
{
using namespace std; // Names of std are visible here
void message() // Function A::message()
{
cout << "Within function A::message()\n";
}
}
namespace B
{
using std::cout; // Declaring cout of std.
void message(void); // Function B::message()
}
void B::message(void) // Defining B::message()
{
cout << "Within function B::message()\n";
}
int main()
{
using namespace std; // Names of namespace std
using B::message; // Function name without braces!
cout << "Testing namespaces!\n";
cout << "\nCall of A::message()" << endl;
A::message();
cout << "\nCall of B::message()" << endl;
message(); // ::message() is hidden because
// of the using-declaration.
cout << "\nCall of::message()" << endl;
::message(); // Global function
return 0;
}
Namespace Using Directive:
Testing namespaces!
Call of A::message()
Within function A::message()
Call of B::message()
Within function B::message()
Call of::message()
Within function ::message()
Using Declaration:
When we use Using Directive, we import all the names in the namespace and they are available throughout the program, that is they have global scope. But With Using Declaration, we import one specific name at a time which is available only inside the current scope.
“
Name Imported with "Using Declaration" can override the name imported with "using Directive"
A using declaration makes an Identifier from a namespace visible in the current scope.
Example:
using code::x; //declaration:
/*Namespace using declaration: */
#include <iostream>
using namespace std;
namespace first_num
{
int i;
}
using namespace first_num; //using declaration:
main()
{
i=2;
cout<<"The value is: "<<i<<endl;
return 0;
}
Namespace Using Declaration:
The value is: 2
In using declaration, we never mention the argument list of a function while importing it, hence if a namespace has overloaded function, it will led to ambiguity.
std Namespace:
All the entities variables, types, constants, and functions) of the standard C++ Library are declared within the std namespace. in most of our these tutorials, we include the following line:
using namespace std;
This introduces direct visibility of all the names of the std namespace into the code. this is done in these tutorials to facilitate comprehension and shorten the length of the example, but many programmers prefer to Qualify each of the elements of standard library used in their programs.
For Example, instead of:
cout<<" Welcome to InfoBrother: "<<endl;
it is common to instead see:
std::cout<<" Welcome to InfoBrother: "<<std::endl;
Whether the elements in the std namespace are introduced with using declarations or are fully Qualified on every user does not change the behaviour or efficiency of the resulting program in any way. it is mostly a matter of style preference, although for projects mixing libraries, explicit tends to be preferred.